Write a program that reproduces itself
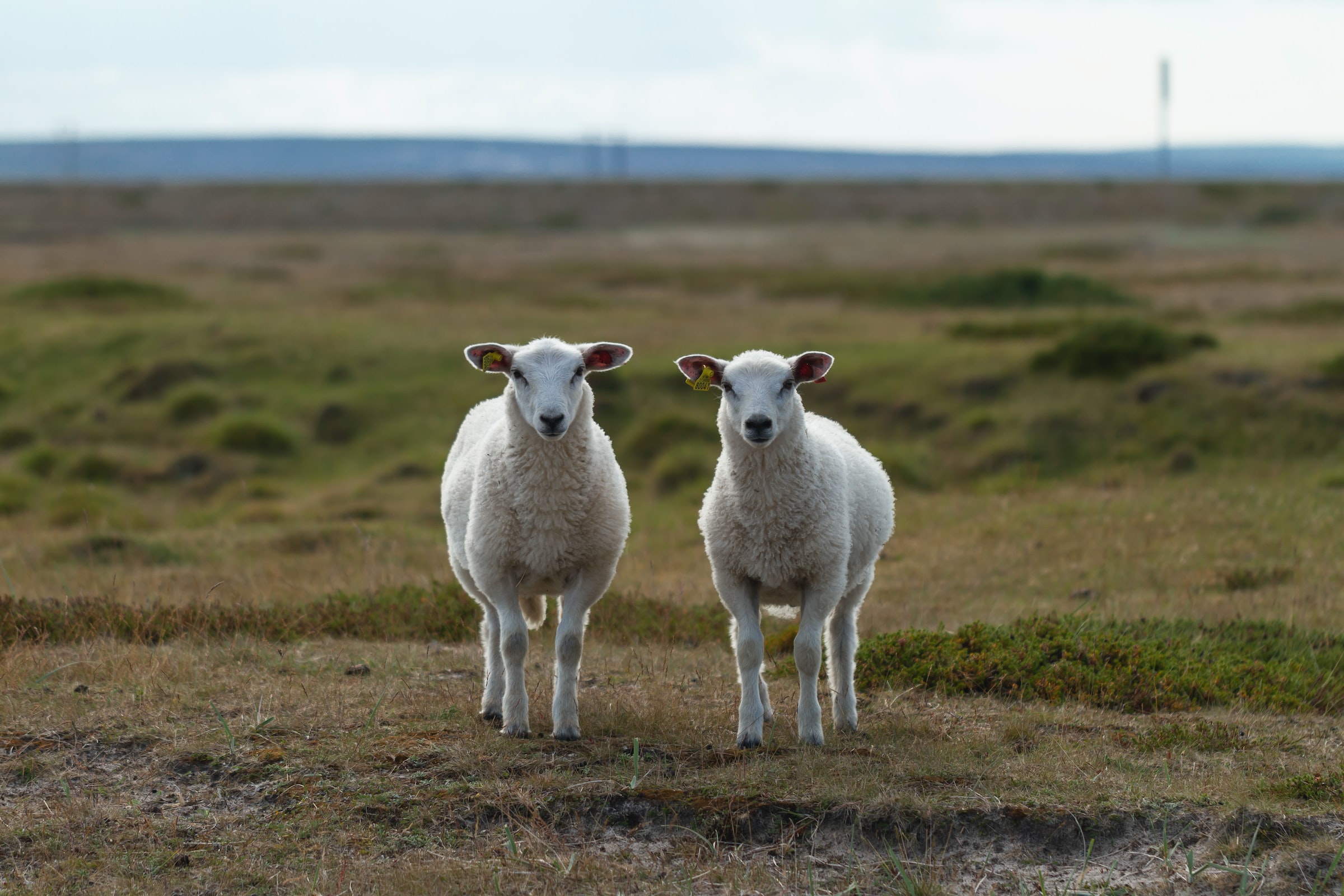
If you like to program, here is a neat little excercise:
Write a program that outputs it's own source code.
It is really fun, you should try it now. For me it is the kind of excercise that won't leave your head once you have written the first line of code.
Spoilers ahead.
How I did it
Python
I am most fluent in python, so that's what I tried first. After some trial and error I had this, which got me pretty close:
var='x'
print(f'{var=}\nprint({var})')
var='x' print(x)
Now all I had to do was to find good value for var
and hope that the escaping would work out.
I ended up with this.
var='print(f"{var=}\\n{var}")'
print(f"{var=}\n{var}")
var='print(f"{var=}\\n{var}")' print(f"{var=}\n{var}")
You can check that this really does output it's own source code by putting it into a file and running (in bash
):
diff code.py <(python code.py) && echo "Success"
This was fun, but I did use a bit of f-string magic. Can we get a similar solution in another language?
sh
Solving a problem in a shell-script is either really easy or really hard. Lets find out:
var='x'
echo -e "var='$var'\n$var"
var='x' x
The first attempt works reasonably well,
but when we replace the string x
with echo -e "var='$var'\n$var"
then we get into trouble.
var='echo -e "var='$var'\n$var"'
echo -e "var='$var'\n$var"
var='echo -e "var= $var"' echo -e "var= $var"
As always when you work with complicated strings in shell scripts, escaping special characters becomes unreasonable difficult.
In the end, I couldn't get it to work.
How to google it
A program that prints it's own source code is called a quine.
You may now google it, or just read the wikipedia article (which is great). I especially enjoyed the cheating section, where it mentioned that an empty program is technically a valid quine, as it outputs nothing when executed.
There is also rosetta code, which lists many solutions that I don't understand and some cheats.